Those are some beautiful diagrams :-)
Ok, so the most important point to realise is that the Marching Cubes algorithm does not actually opaerate on individual voxels, but rather it processes groups of eight voxels at at time. A group of eight voxels is called a 'cell' (or at least that is what I call it).
When processing a cell the algoithm generates a number of vertices which make up the triangles. Each vertex lies exactly on an edge of the cell, and so is between exactly two voxels. Here's the image from Wikipedia:
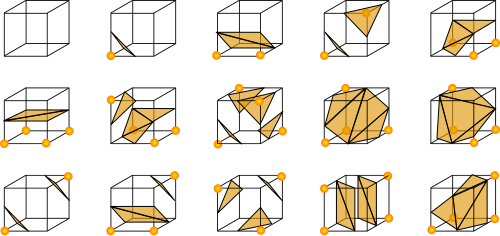
In that image each box is a cell, and the eight corners are the eight voxels. A voxel can be solid or empty (indicated by the presence or absence of the yellow dot) and a vertex always lies between two voxels.
So, given two voxels with a vertex between them, how do we decide exactly where the vertex should go? The easiest thing is to place it in the middle (as shown in that figure) but to get smooth terrain is is often useful to place it nearer to one of the voxels than the other.
If your two voxels have densities of 0 and 255, and your 'threshold' value is 128 then the vertex will be placed in the middle. On the other hand, if the voxel values are 0 and 130 the the vertex will be placed very close to to voxel with a value of 130. In other words, the algorithm takes the two voxel values and determines where the threshold value would fall between them.
Clonkex wrote:
I forgot that the main reason I posted this was because I was confused as to how to use densities if you need the voxel to contain more than just an integer. What if I need a voxel to contain a struct with several other variables in it? What controls the density in that case?
It's quite advanced (needs some C++ template knowledge) but you can provide a custom marching cubes controller. The default version contains some documentation and is here:
https://bitbucket.org/volumesoffun/poly ... ?at=masterHope that helps :-)